================= Following =======================
Let's implement mainPage, which is visible when we jump from the loading page.
First of all, I recommend you to get a basic knowledge with the xoppa blog on basic implementation.
https://xoppa.github.io/blog/basic-3d-using-libgdx/
Basic 3D using libGDX | blog.xoppa.com
This tutorial guides you in using the basics of the 3d api LibGDX offers.
xoppa.github.io
I'll implement it similar to the implementation page for three.js in my blog. I'll add a light, add a camera control, a sphere, a cube, and a line to determine its size.
Below is the screen configuration.
https://cyjses.tistory.com/33?category=833857
Three.js - 충돌처리(collision, bullet engine) - part2
불릿 엔진의 모든 설명을 하기에는 내용상으로 너무 방대하고, 또 저의 실력이 그정도 되지 않습니다. 대신 LibGdx에서 bullet engine wrapper 에 관한 매우 좋은 레퍼런스를 이전 장에서 소개했습니다. LibGdx를..
cyjses.tistory.com
libgdx, unlike threejs, does not have some helpers. And the basic model implementation may seem a bit difficult. Please take a look at the source and create a new class to organize your own implementation rhythm.
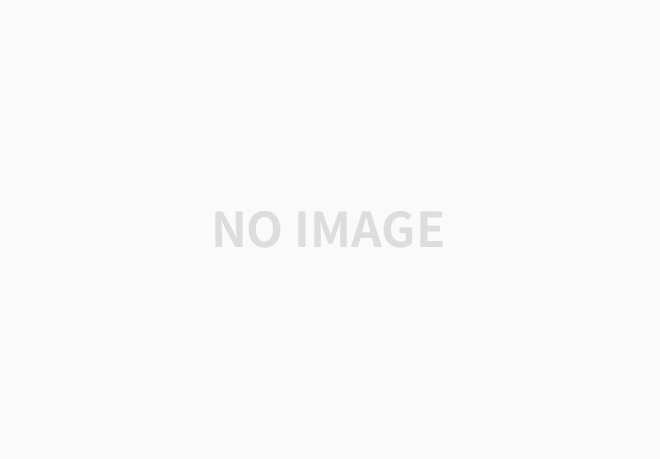
I'll only explain what's added in the basic document.
1. Help class to operate camera with mouse.
2. I added a light, and added two ambient light, ambient light and two directional light. Add it to the environment, and specify this environment when rendering to render the lighted screen.
3. modelBuilder-libgdx creates a basic model first and then creates an instance based on it.
modelBuilder is a class that creates basic shapes. There are two ways to create it.
The first method is to create a cube or sphere consistently as in the case below. This method only generates the shape in the specified way.
The second way is to create a model by combining several shapes with the meshPartBuilder as an intermediate, as in step 4. To do this, start with modelBuilder.begin (), add several shapes in the middle with meshPartBuilder, and then do modelBuilder.end (). The model is returned as the return value.
Number 4 is creating a grid to help you measure size. This part should be automated by the programmer.
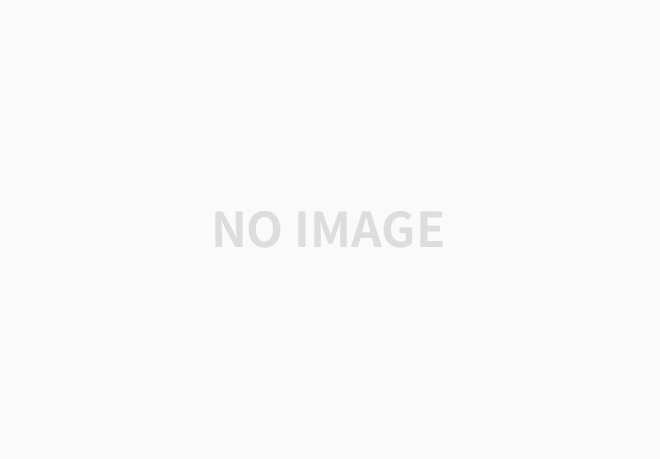
Step 5 adds three lines that tell the axis that modelBuilder is not finished.
Then call modelBuilder.end () to get the work done.
As mentioned above, 6 creates a model using the specified format, such as createBox and createSphere.
Material 7 and attribute 7 specify the rendering texture, color, and format. This part also has a lot of content, so I will not discuss it here. Find the libgdx document separately. You can see the color assignment.
8, 9-> Rendering.
The resulting screen looks like this:
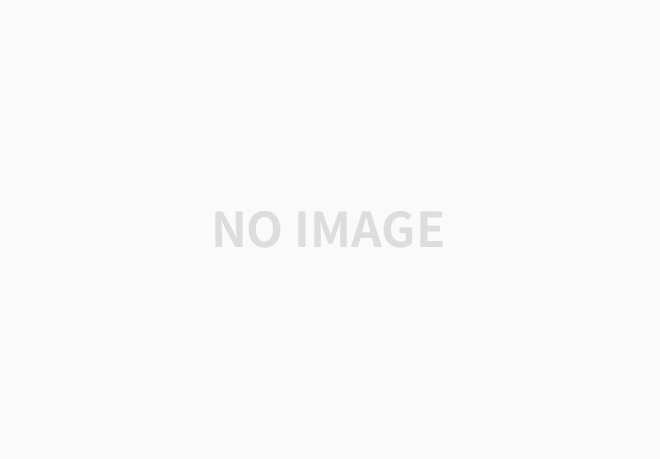
Finally, let's look at dispose.
dispose is to free memory. As you know java automatically runs the garbage collector. However, such game engines use too large data and force them to be released manually. In other words, some resources have to dispose directly.
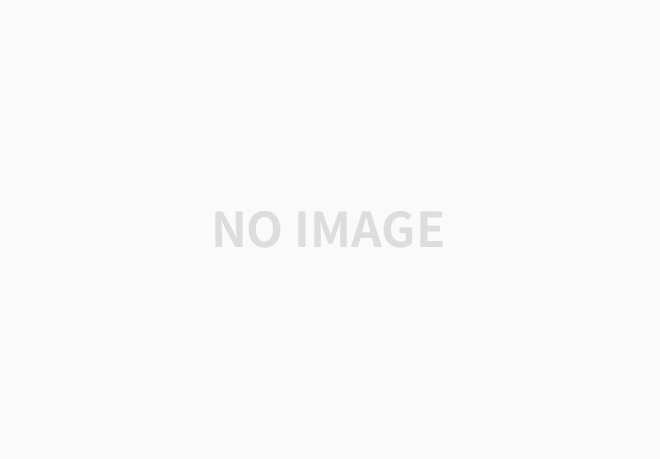
Dispose here is modelbatch and model. You can understand that this class has a lot of resources. Knowing what resources to dispose is to test whether you have a dispose as a method. The libgdx documentation teaches you the overall kind. And as I'll mention later, scene2d, which handles fonts and images, should also dispose most elements. This is covered in that section. Here is the libgdx document:
https://github.com/libgdx/libgdx/wiki/Memory-management
libgdx/libgdx
Desktop/Android/HTML5/iOS Java game development framework - libgdx/libgdx
github.com
Now let's finish the loading page, write the part that goes to the main page, and complete the project.
Attach source code containing only the following resources and sources.
True, and change GLOBAL.where to mainPage to run the above page.
Will continue in the next post.
=========================================================================
'LibGdx' 카테고리의 다른 글
libgdx-collision handling (collision, bullet engine) (0) | 2019.11.18 |
---|---|
libgdx-loading page implementation 5 (1) | 2019.11.17 |
libgdx-loading page implementation 3 (skin, font) (0) | 2019.11.16 |
libgdx-loading page implementation 2 (0) | 2019.11.12 |
libgdx-loading page implementation, (using skin) (0) | 2019.11.12 |
댓글