libgdx provides a bullet engine wrapper by default. If checked at initial setting, bullet library is added. Otherwise, it is added to build.gradle.
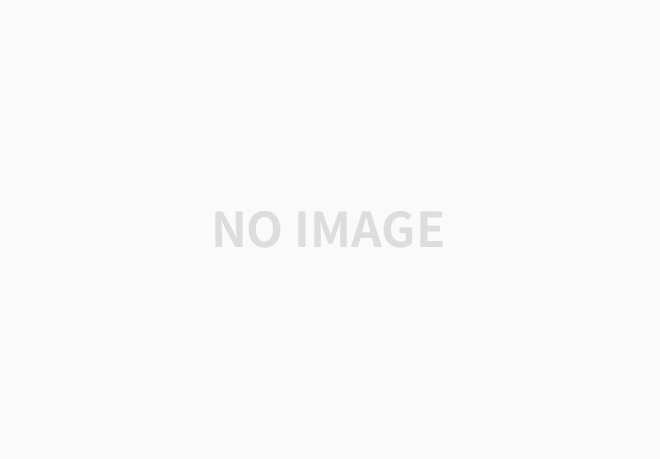
Or add the following to your build.gradle
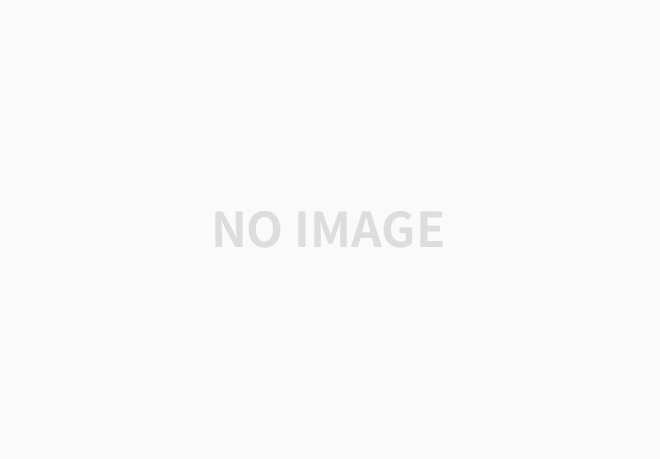
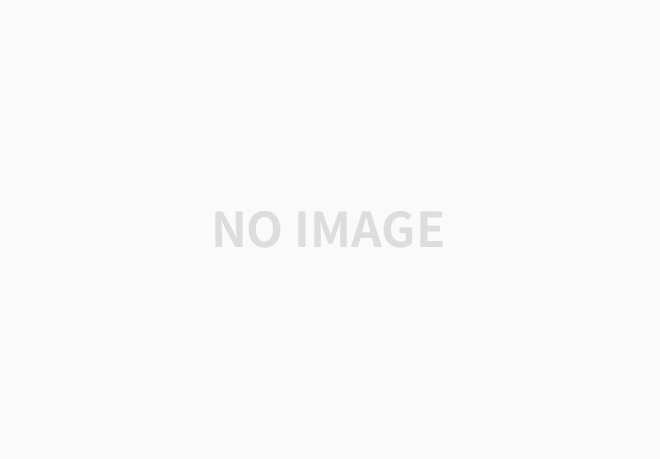
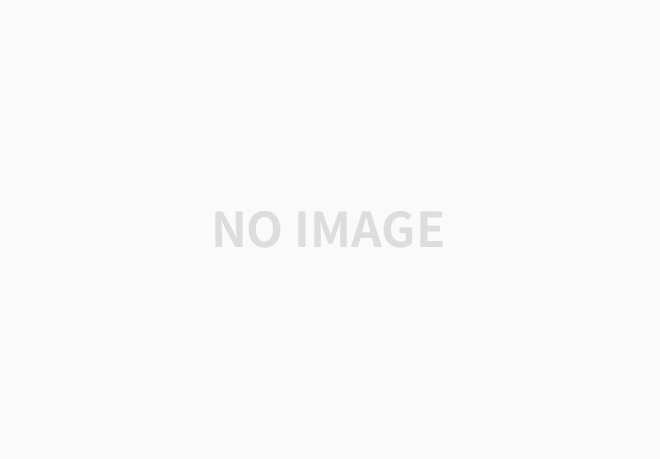
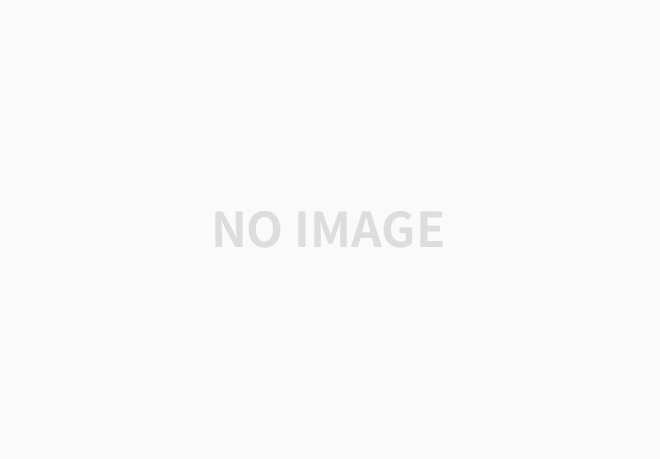
There are some good documentation on using the libgdx bullet engine.
https://xoppa.github.io/blog/using-the-libgdx-3d-physics-bullet-wrapper-part1/
Using the libGDX 3D physics Bullet wrapper - part1 | blog.xoppa.com
Many 3D games require some sort of collision detection between 3D objects. Sometimes it's possible to do this with some basic math and bounding boxes and...
xoppa.github.io
The documentation is in the order of collision world-> dynamic world-> motion state. The implementation in this post will even use motion states. The functionality is almost identical to the implementation in three.js on the blog. Since the description is so detailed in the xoppa blog above, the details are reduced, and the description in my blog is more detailed in three.js. please note.
https://cyjses.tistory.com/33?category=833857
Three.js - 충돌처리(collision, bullet engine) - part2
불릿 엔진의 모든 설명을 하기에는 내용상으로 너무 방대하고, 또 저의 실력이 그정도 되지 않습니다. 대신 LibGdx에서 bullet engine wrapper 에 관한 매우 좋은 레퍼런스를 이전 장에서 소개했습니다. LibGdx를..
cyjses.tistory.com
That's how I'm going to implement it this way.
1. Visually identify the collision object.
2. Basically you can add mixed objects.
========================================================================
Let's add a CollisionElement that returns the most basic shapes like boxes, spheres, triangular pyramids and cylinders. The implementation is the same as three.js on the blog.
Implemented as Factory Class.
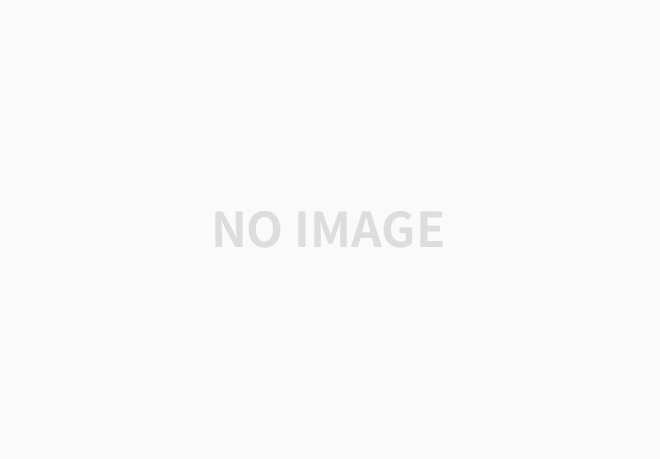
1. bounding is a visual frame created by lines. Collision puts the shape of a bullet that represents the actual collision object.
2. boudingBuiler-I explained ModelBuilder in the previous post. There is a way to create a single basic shape and a combination of multiple shapes with the meshPartBuilder.
3. build_count increments as each conflict shape is added to each instance. As we saw in 4, at zero, the first time we declare boundingbuilder.begin () to declare that we are creating a mesh part.
5. Call node () before adding one mesh part.
Steps 3 through 5 are common when using meshPartBuilder.
6,7-We are creating a model for bounding and a shape for collision, respectively.
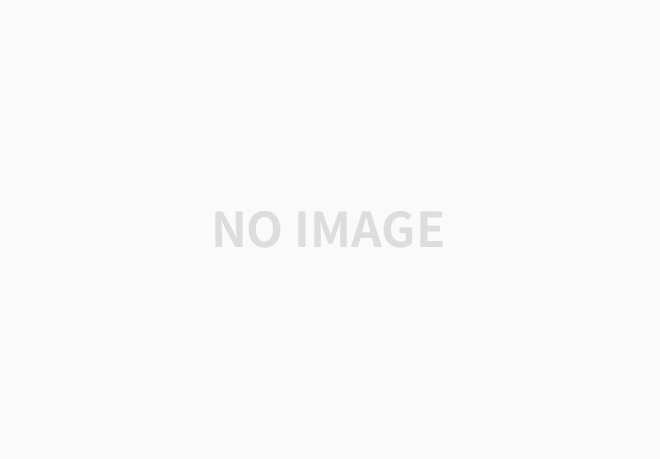
In the case of a collation shape, you need to add it to the compoundShape every time it is created. The bounding line model doesn't have to do that, add nodes one by one with the meshPartBuilder, and call end () when you're done. The end () method executes that process.
=============================================
Next, we will create a PrimitiveModel class that will return a model instance and a rigid body.
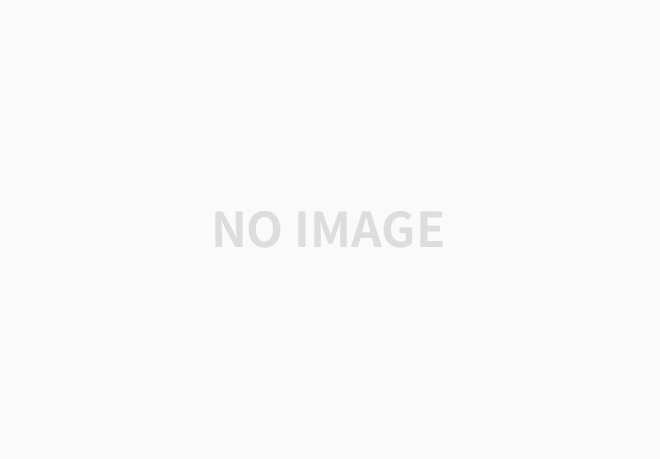
1. An inner class that extends motionState.
2. You can see that we have two fileds: bounding_line and collision_body.
3. shapes is an array that stores a link to each shape you create in the CollsionElement. So use it to dispose when you're done.
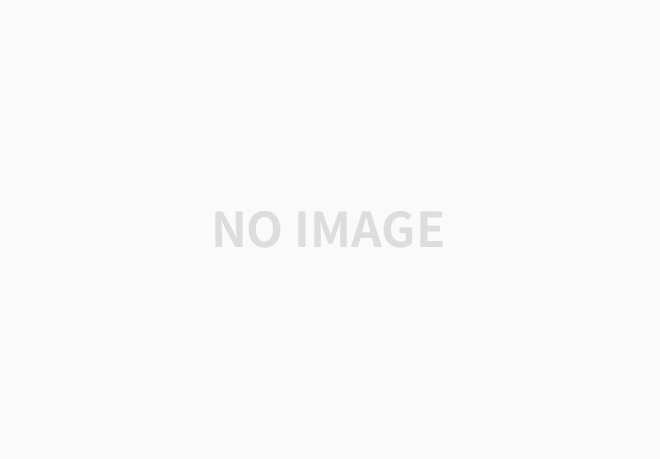
1. The original model you want to show.
2. Create a collision shape from the CollisionElement you created earlier.
3. Change the position value of the returned CollisionElement. There is no change here.
4. Add it to the CompoundShape.
5. Save the link of the collision shape for dispose.
Steps 2 through 5 can be added and repeated several times to create complex shapes.
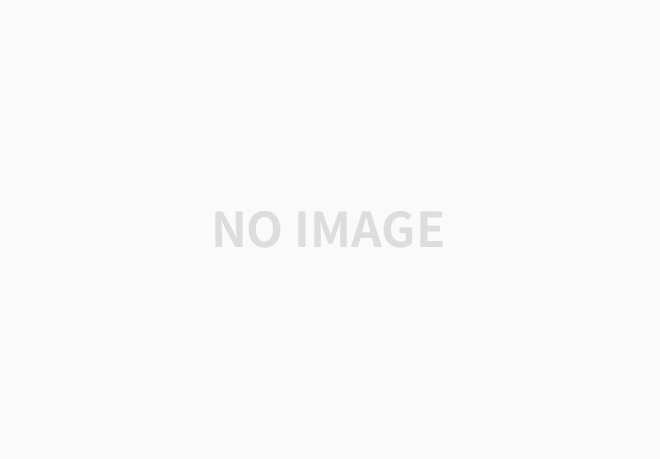
1. In this way, model the bounding line that has been composited.
2. This is the part to set the inertia force.
3. Create a rigidbody.
4. Create a motion state.
==================================================================
We have not yet set the method of moving the object due to the motion state. And no dynamic world. But basic visual tools and rigid bodies have been created. Let's check the execution result first.
Now let's create a main page for the output.
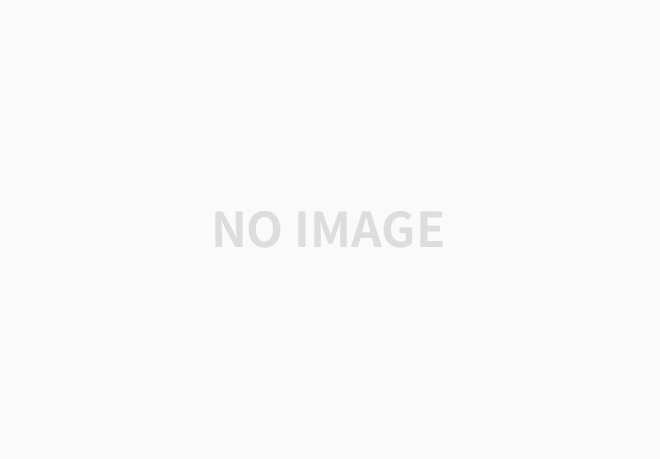
In the example in the previous post, change the following: Rather than using modelInstances one by one, we use an array to collect them according to their characteristics. This allows you to send an array when rendering.
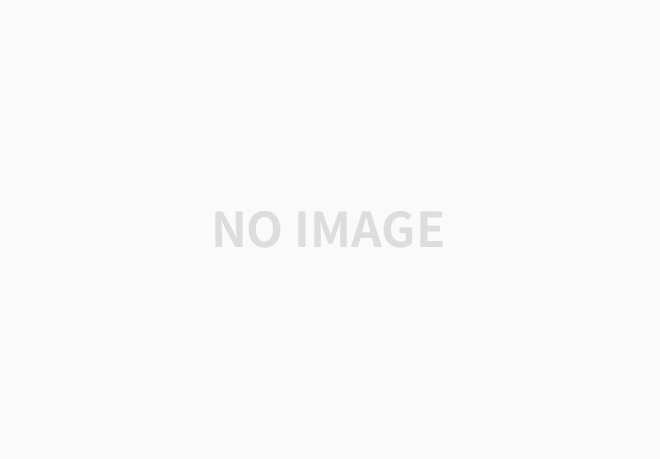
1. Now let's render the grid and axe to others.
2. You need to run Bullet.init () first to start the bullet engine.
3. The previous example is shown as a PrimitiveModel. Add the actual modelInstance and bouding lines to instances and others respectively.
4. Changed the rendering method like this.
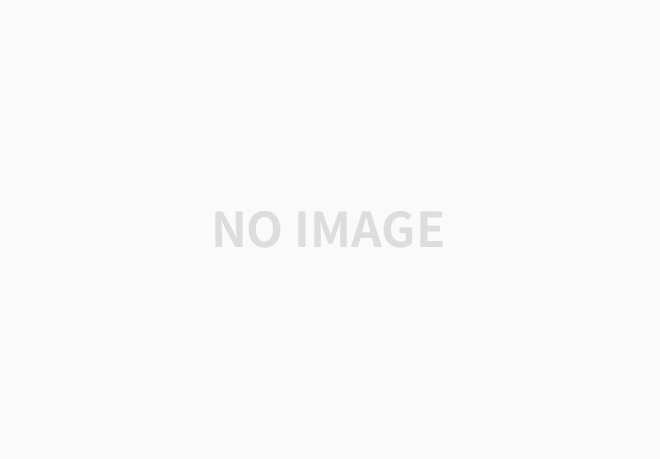
============== leads to the next post =============
'LibGdx' 카테고리의 다른 글
libgdx-collision handling 3 (collision, bullet engine) (0) | 2019.11.18 |
---|---|
libgdx-collision handling 2 (collision, bullet engine) (0) | 2019.11.18 |
libgdx-loading page implementation 5 (1) | 2019.11.17 |
libgdx-loading page implementation 4 (basic 3d scene configuration) (0) | 2019.11.16 |
libgdx-loading page implementation 3 (skin, font) (0) | 2019.11.16 |
댓글