It's too big for me to explain all of the bullet engines, and my skills are not that good. Instead, LibGdx introduced a very good reference to the bullet engine wrapper in the previous chapter. If you don't know LibGdx, don't be too shy and have fun reading. If you come to the level of the program anyway, there is no easy content.
https://xoppa.github.io/blog/using-the-libgdx-3d-physics-bullet-wrapper-part1/
Using the libGDX 3D physics Bullet wrapper - part1 | blog.xoppa.com
Many 3D games require some sort of collision detection between 3D objects. Sometimes it's possible to do this with some basic math and bounding boxes and...
xoppa.github.io
Once you've studied the reference, don't get too caught up in the parts you don't understand.
I'm going to implement it this way.
1. Visually identify the collision object.
2. Basically you can add mixed objects.
Meaning 1 means that if there is an object like the one below, it draws a border to know its collision range.
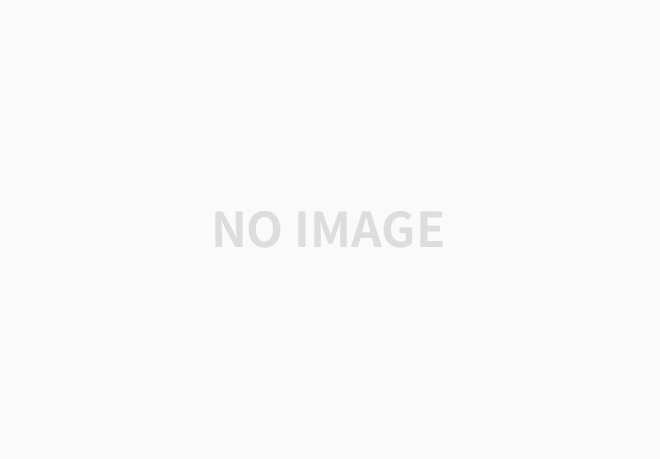
Meaning 2 means that we will use compoundShape to cover not only the basic shape but also various shapes.
compoundShape can have multiple default shapes. Then you can combine spheres and hexagons to handle complex shapes.
The implementation does not have to be this way.
First, let's implement a simple object provided by three js.
Let's create a class template called PrimitiveModel.js. (Note that I wrote the model as model, but it is implementation specific. It is just a name.)
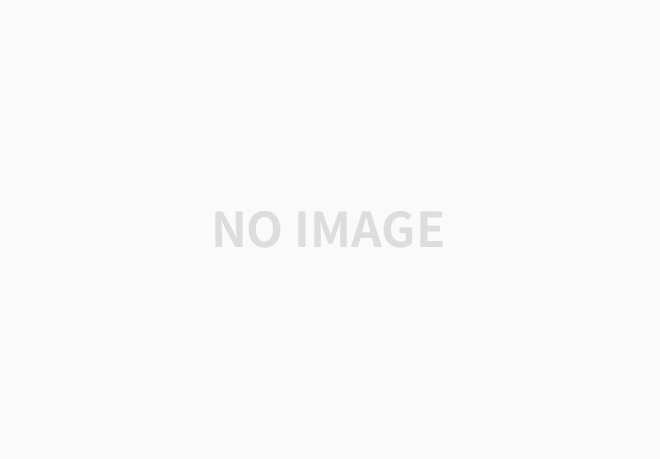
This is the basic framework of a class called PrimitiveModel that inherits SkinnedMesh with an es5 shape.
But since it is the es6 era, I will write it as es6.
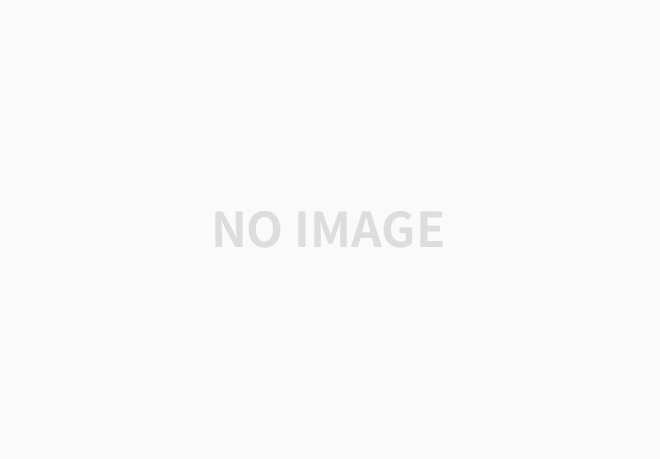
Let's put one sphere and one box in the mainPage as a primitiveModel.
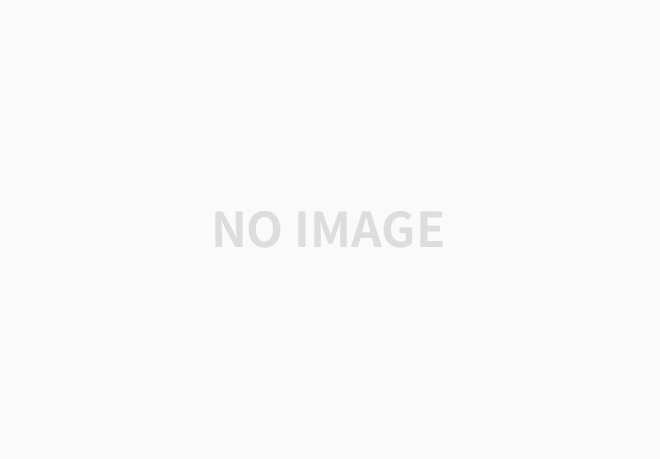
At the top, import PrimitiveModel.
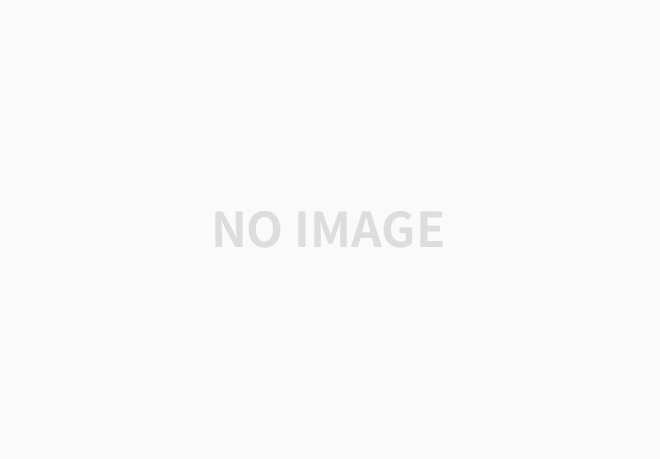
And if you add it like this, the output looks like the first picture in this post. It's nothing unusual.
===========================================================================
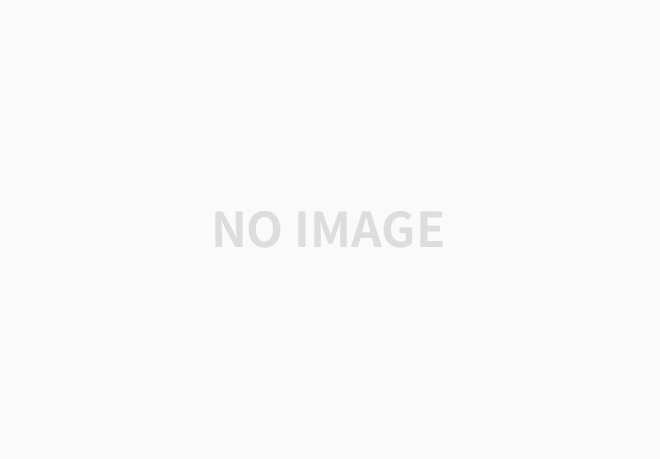
Let's add another object in this state. The name is CollisionElement. The basic framework of the implementation is to return the most basic shapes such as boxes, spheres, triangular pyramids, and cylinders, and to call each from PrimitiveModel and use it for each individual model.
bounding is a frame created by line. Collision puts the shape of a bullet that represents the actual collision object.
Actual javascript does not declare the privite variable of the class. In other words, bounding or collision can be used immediately. In other words, you don't have to put an empty variable like I did. I've allocated an empty object {} for clarity. Please do not make a mistake.
The second part of the static expression is similar to the static method in java. In fact, after declaring a class in javascript, you can use it like a static method by associating a method with the class name this way. If you have another way, you can use that.
===========================================================================
Next, let's summarize the basic meshes to use.
BybtBoxShape => BoxGeometry
2. btSphereShape => SphereGeometry
3. btCylinderShape => CylinderGeometry
-> Rectangle, sphere, cylinder shape respectively. On the right is the corresponding mesh geometry in three.js.
4. btConvexHullShape
-> This function creates a convex shape. Adding a point creates a shape based on the convex shape along that point.
Let's code inside CollisionElement.make.
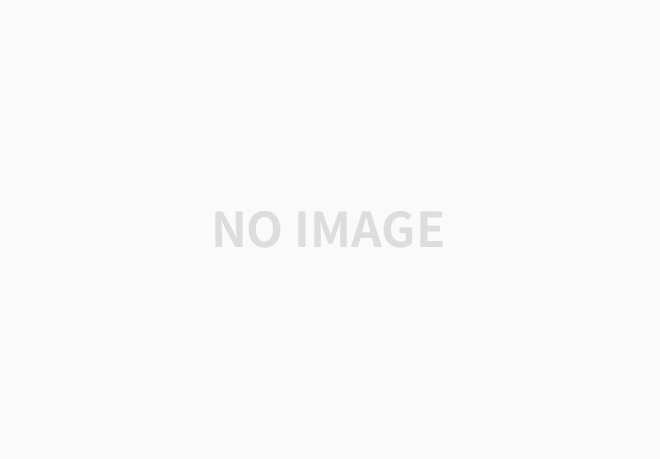
Line 15 is called CollisionElement.make ("box", ...) this way and it will be the first argument. Name
Line 16 receives the required numbers after the 'name' argument, which depends on the shape.
line 19: bounding_geometry, geometry varies depending on the type of call, defines a variable that accepts it
line 20: bounding_material is commonly red and wireframe.
Then branch according to the type of name,
The number of numbers required depends on the type of name. Check it out,
} else if (name == "box") {
if (point.length != 3) throw exception;
bounding_geometry = new THREE.BoxGeometry( point[0], point[1], point[2] );
node = new THREE.Mesh( bounding_geometry, bounding_material );
newElement.bounding = node;
newElement.collision = new Ammo.btBoxShape(new Ammo.btVector3(point[0] / 2, point[1] / 2, point[2] / 2));
For example, in case of "box", make the shape in bounding / collision variable as above.
There are four custom implementations of "diamond", "sadari", "arrow", and "(free form)".
For example, "sadari"-(Trapezoid) is implemented like this.
If CollisionElement.make ("sadari", width_up, width_down, height, offset, depth_up, depth_down, offset1, offset2, offset3, offset4); It is like this.
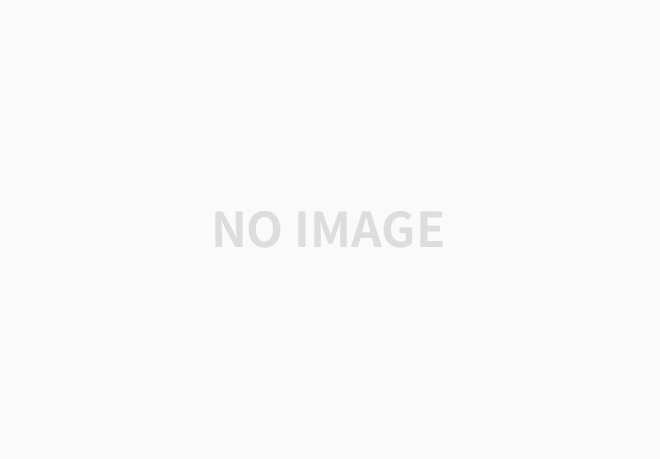
offset is the offset by which width_up moves left and right, offset1 / offset2 / offset3 / offset4 means the offset by which depth1 / depth2 / depth3 / depth4 can move back and forth.
If you implement like this
return_value = CollisionElement.make ("box", 2,2,2);
If you receive a return_value with visual bounding and collision collision.
===========================================================================
Leads to the next chapter
'Three.js' 카테고리의 다른 글
Three.js-collision and bullet engine, ammo -part4 (0) | 2019.10.22 |
---|---|
Three.js-collision, bullet engine, ammo -part3 (0) | 2019.10.22 |
Three.js-collision and bullet engine, ammo -part1 (2) | 2019.10.15 |
Three.js - Animation system (1) | 2019.10.15 |
Three.js-key event (0) | 2019.10.10 |
댓글