Bullet provides three easy ways to ensure that only certain objects collide with each other: masks, broadphase filter callbacks and nearcallbacks. It is worth noting that mask-based collision selection happens a lot further up the toolchain than the callback do. In short, if masks are sufficient for your purposes, use them; they perform better and are a lot simpler to use. Of course, don't try to shoehorn something into a mask-based selection system that clearly doesn't fit there just because performance may be a little better.
Bullet은 마스크, 광대역 필터 콜백 및 니어 콜백이라는 특정 오브젝트 만 서로 충돌하도록하는 세 가지 쉬운 방법을 제공합니다. 마스크 기반 충돌 선택이 콜백보다 도구 체인에서 훨씬 더 많이 발생한다는 점은 주목할 가치가 있습니다. 간단히 말해서 마스크가 목적에 충분하다면 사용하십시오. 성능이 더 우수하고 사용하기가 훨씬 간단합니다. 물론 성능이 조금 나아질 수 있다고해서 거기에 확실히 맞지 않는 마스크 기반 선택 시스템에 무언가를 넣어서는 안됩니다.
Filtering collisions using masks
Bullet supports bitwise masks as a way of deciding whether or not things should collide with other things, or receive collisions.
Bullet은 사물이 다른 사물과 충돌해야하는지 또는 충돌을 받아야하는지 여부를 결정하는 방법으로 비트 마스크를 지원합니다.
int myGroup = 1;
int collideMask = 4;
world->addCollisionObject(object,myGroup,collideMask);
During broadphase collision detection overlapping pairs are added to a pair cache, only when the mask matches the group of the other objects (in needsBroadphaseCollision)
광 위상 충돌 감지 동안 겹치는 쌍은 쌍 캐시에 추가되며 마스크가 다른 객체 그룹과 일치 할 때만 (needsBroadphaseCollision에서)
bool collides = (proxy0->m_collisionFilterGroup & proxy1->m_collisionFilterMask) != 0;
collides = collides && (proxy1->m_collisionFilterGroup & proxy0->m_collisionFilterMask);
If you have more types of objects than the 32 bits available to you in the masks, or some collisions are enabled or disabled based on other factors, then there are several ways to register callbacks to that implements custom logic and only passes on collisions that are the ones you want:
마스크에서 사용할 수있는 32 비트보다 더 많은 유형의 객체가 있거나 다른 요인에 따라 일부 충돌이 활성화 또는 비활성화 된 경우 사용자 정의 논리를 구현하고 다음과 같은 충돌에만 전달하는 콜백을 등록하는 몇 가지 방법이 있습니다. 당신이 원하는 것 :
Filtering Collisions Using a Broadphase Filter Callback
One efficient way is to register a broadphase filter callback. This callback is called at a very early stage
in the collision pipeline, and prevents collision pairs from being generated.
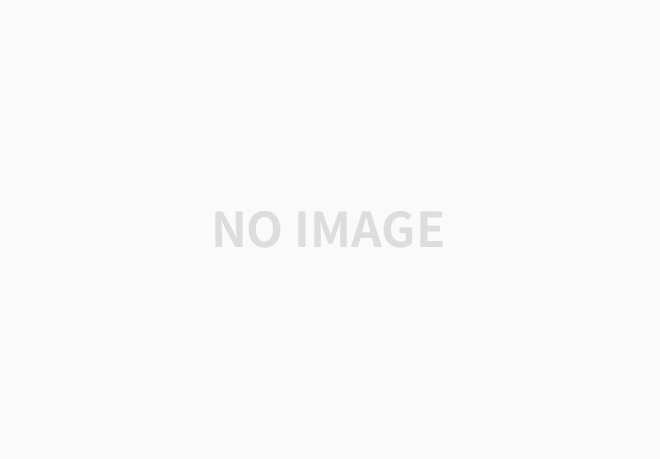
And then create an object of this class and register this callback using:
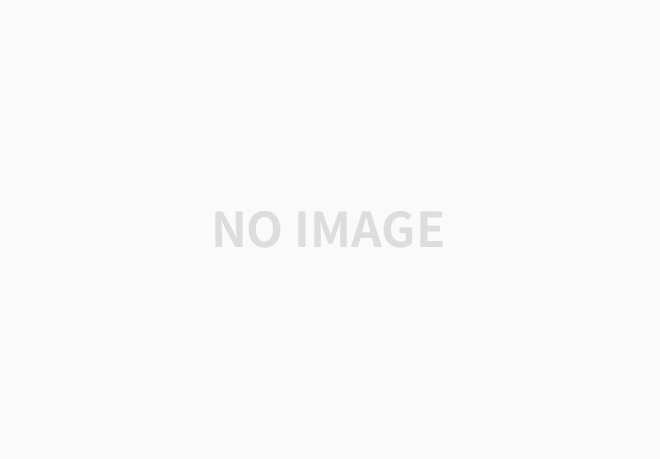
Filtering Collisions Using a Custom NearCallback
Another callback can be registered during the narrowphase, when all pairs are generated by the broadphase. The btCollisionDispatcher::dispatchAllCollisionPairs calls this narrowphase nearcallback for each pair that passes the 'btCollisionDispatcher::needsCollision' test. You can customize this nearcallback:
모든 쌍이 브로드 페이즈에 의해 생성되는 좁은 페이즈 동안 다른 콜백을 등록 할 수 있습니다. btCollisionDispatcher :: dispatchAllCollisionPairs는 'btCollisionDispatcher :: needsCollision'테스트를 통과하는 각 쌍에 대해이 협소 단계 니어 콜백을 호출합니다. 이 니어 콜백을 사용자 정의 할 수 있습니다.
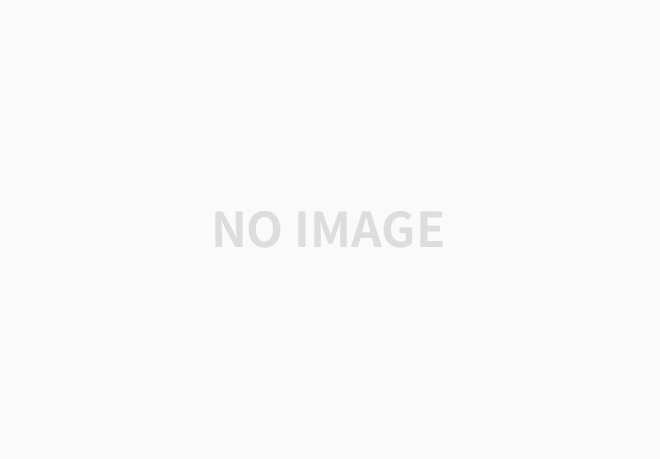
Deriving your own class from btCollisionDispatcher
For even more fine grain control over the collision dispatch, you can derive your own class from btCollisionDispatcher and override one or more of the following methods:
충돌 디스패치를 더욱 세밀하게 제어하려면 btCollisionDispatcher에서 고유 한 클래스를 파생하고 다음 메서드 중 하나 이상을 재정의 할 수 있습니다.
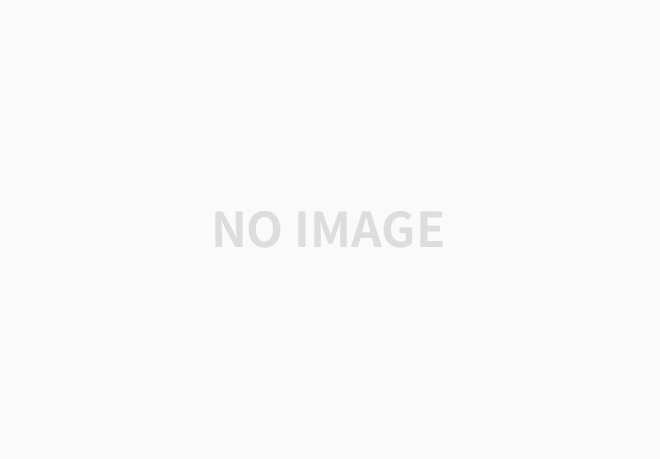
'Bullet Physics' 카테고리의 다른 글
Constraints (0) | 2020.12.03 |
---|---|
Rigid Body Dynamics (0) | 2020.12.02 |
Bullet Collision Detection (0) | 2020.12.02 |
Basic Data Types and Math Library (0) | 2020.12.02 |
Rigid Body Physics Pipeline (0) | 2020.12.02 |
댓글