Install sdk from the Jmonkey wiki.
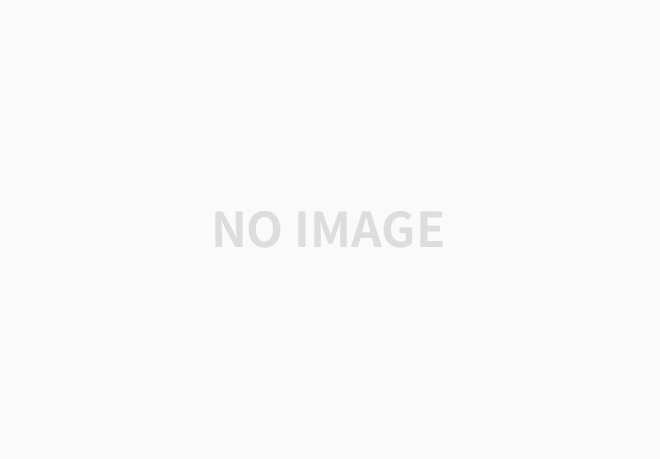
Create a new project with new Project-> JME3-> BasicGame.
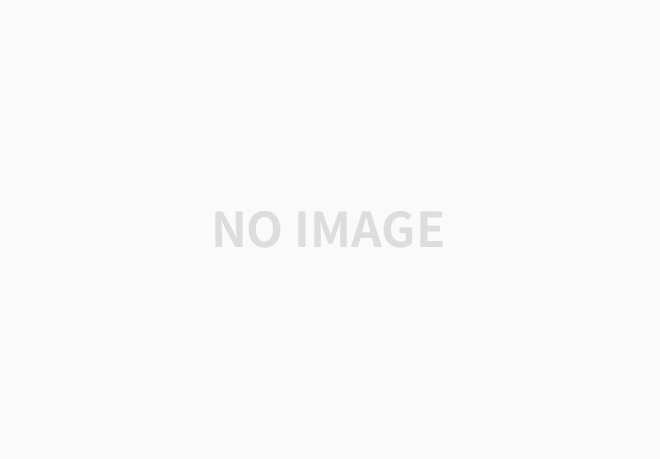
Press the arrow to run
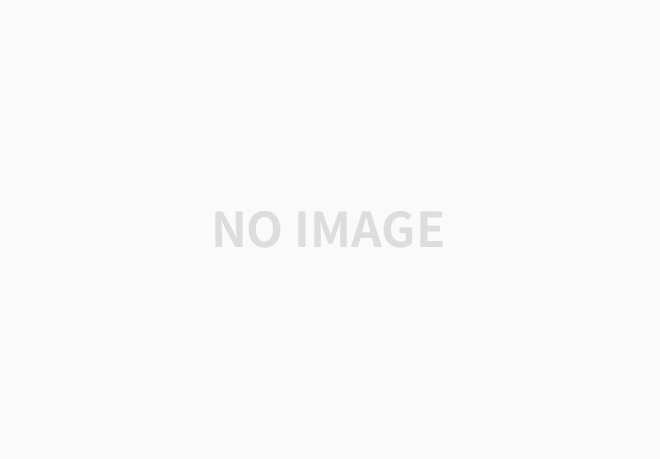
It's a bit unnatural because it takes up all the input. I think it's because Jmonkey started a program for desktop. First change the input.
For initial execution, please refer to the following tutorial 1-3.
https://www.youtube.com/watch?v=h6Xl3MRjMLM
Right click on mygame in Source Packages to add new package and start it.
Source Packages is the same as the src of the actual folder.
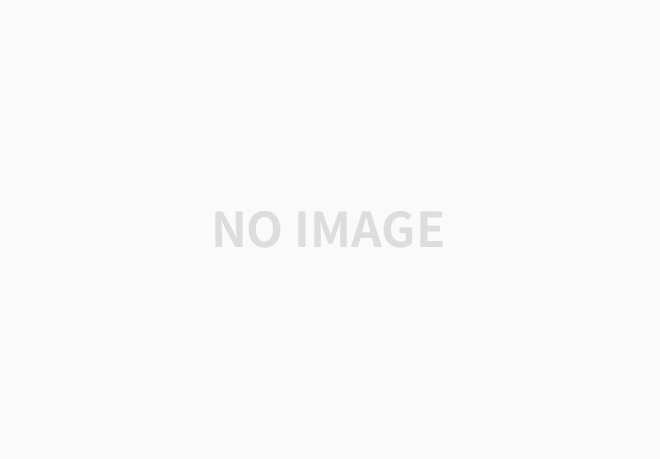
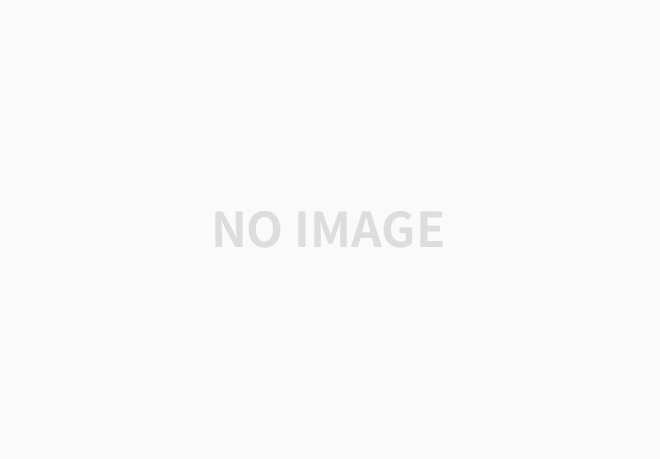
Create a package called newpackage.state and create a Level01State.java file as follows:
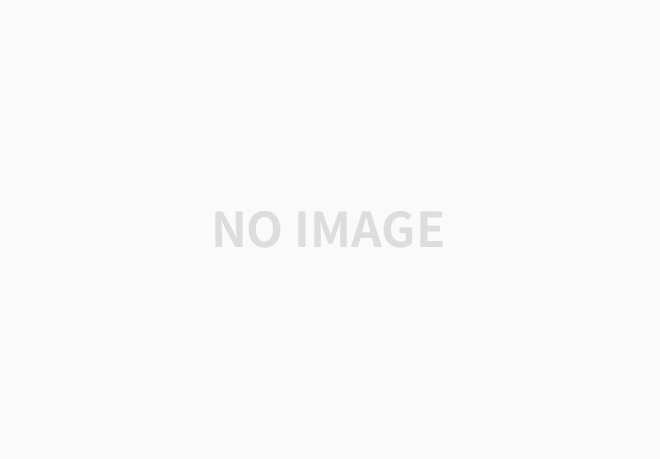
Modify the source as follows:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
|
public class Level01State extends AbstractAppState{
private final Node rootNode;
private final Node localRootNode = new Node("Level 1");
private final AssetManager assetManager;
private final InputManager inputManager;
private final FlyByCamera flyByCamera;
private final Camera camera;
private ChaseCamera chaseCamera;
public Level01State(SimpleApplication app) {
rootNode = app.getRootNode();
assetManager = app.getAssetManager();
inputManager = app.getInputManager();
flyByCamera = app.getFlyByCamera();
camera = app.getCamera();
}
@Override
public void initialize(AppStateManager stateManager, Application app) {
super.initialize(stateManager, app); //To change body of generated methods, choose Tools | Templates.
rootNode.attachChild(localRootNode);
inputManager.addListener(actionListener);
flyByCamera.setEnabled(false);
chaseCamera = new ChaseCamera(camera, localRootNode, inputManager);
Box b = new Box(1, 1, 1);
Geometry geom = new Geometry("Box", b);
Material mat = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md");
mat.setColor("Color", ColorRGBA.Blue);
geom.setMaterial(mat);
localRootNode.attachChild(geom);
}
private final ActionListener actionListener = new ActionListener() {
@Override
public void onAction(String name, boolean keyPressed, float tpf) {
}
};
@Override
public void cleanup() {
rootNode.detachChild(localRootNode);
super.cleanup();
}
@Override
public void update(float tpf) {
}
}
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
public class Main extends SimpleApplication {
public static void main(String[] args) {
Main app = new Main();
app.start();
}
@Override
public void simpleInitApp() {
stateManager.attach(new Level01State(this));
}
@Override
public void simpleUpdate(float tpf) {
//TODO: add update code
}
@Override
public void simpleRender(RenderManager rm) {
//TODO: add render code
}
}
|
cs |
As soon as you start, the main screen just throws you into Level01State.
Level01State extends AbstractAppState, which can be thought of as one page. When there is a scene change,
The stateManager is expected to be attached and detached. Jmonkey is said to recognize all elements (scenes, characters) as "Nodes". The top-level "Node" becomes the first executor "Main", which extends "SimpleApplication". You can see that "SimpleApplication" is a node with RootNode, AssetManager, InputManager, FlyByCamera, Camera, ... etc. Level01State is just getting from the first run.
And AbstractAppState creates a page, which itself does update (). This is a small rendering part, and you can think of simpleRender as the whole unified rendering in Main.java.
I've disabled flyCam and connected chaseCam.
chaseCamera = new ChaseCamera (camera, localRootNode, inputManager);
The second parameter is the target to be chased, just now none, so we hooked up to localRootNode. Because every element is a Node. Here we can just think of localRootNode as a scene.
And I originally added the basic code.
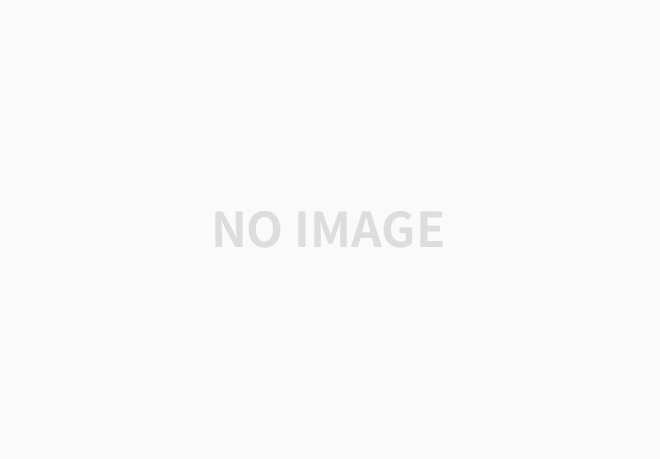
It's a little plain running screen.
Next, let's attach a basic physics engine.
========================================
Project Assets -> Scenes -> right click -> others -> Scene -> Empty JME3 Scene
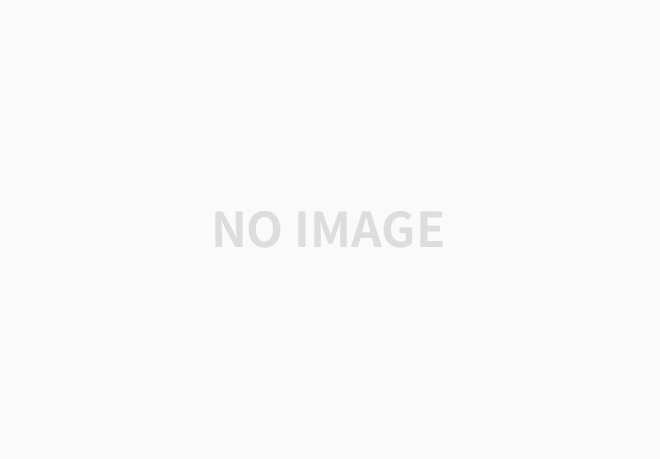
Level1.j3o -> right click -> Edit in SceneComposer
SceneExplorer : Scene Root -> right click -> Add Spacial -> Primitive -> Box & Sphere
Sphere select : Sphere - Properties LocalTraslation -> (0, 5, 0)
Box select : "add collision shape" click -> PhysicsControl - Properties -> mass : 0.0
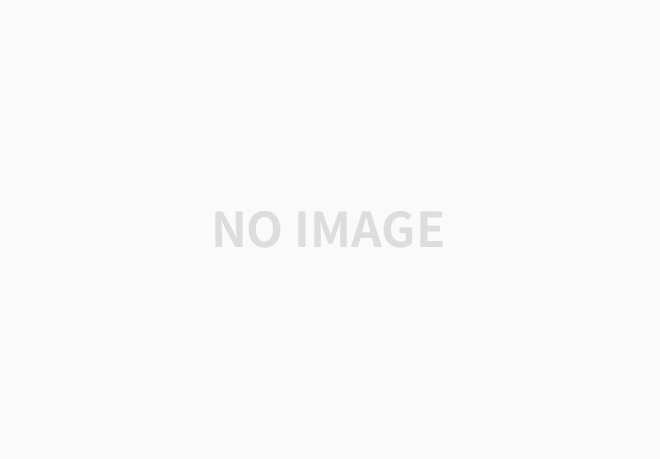
Three.js and libgdx in my blog have a very similar structure. Think of the box as solid ground and drop the sphere.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
|
private final Node rootNode;
private final Node localRootNode = new Node("Level 1");
private final AssetManager assetManager;
private final InputManager inputManager;
private final FlyByCamera flyByCamera;
private final Camera camera;
private ChaseCamera chaseCamera;
private BulletAppState bulletAppState;
private CharacterControl sphereControl;
@Override
public void initialize(AppStateManager stateManager, Application app) {
super.initialize(stateManager, app); //To change body of generated methods, choose Tools | Templates.
rootNode.attachChild(localRootNode);
inputManager.addListener(actionListener);
flyByCamera.setEnabled(false);
chaseCamera = new ChaseCamera(camera, localRootNode, inputManager);
bulletAppState = new BulletAppState();
bulletAppState.setDebugEnabled(true);
stateManager.attach(bulletAppState);
Spatial scene = assetManager.loadModel("Scenes/Level1.j3o");
localRootNode.attachChild(scene);
Spatial box = localRootNode.getChild("Box");
bulletAppState.getPhysicsSpace().add(box.getControl(RigidBodyControl.class));
Spatial sphere = localRootNode.getChild("Sphere");
SphereCollisionShape sphereShape = new SphereCollisionShape(1);
sphereControl = new CharacterControl(sphereShape, 1.0f);
sphereControl.setGravity(new Vector3f(0, -10f, 0));
sphere.addControl(sphereControl);
bulletAppState.getPhysicsSpace().add(sphereControl);
}
|
cs |
Modify the source as above.
Although modified in the form of the Jmonkey SDK, the basic flow is no different from the collision handling of three.js or libgdx.
It seems to set the dynamics world only with the declaration of BulletAppState. setDebugEnabled will show the collision wire-frame.
Box adds PhysicsControl to SceneComposer's visual tool, and Sphere adds code.
bulletAppState.getPhysicsSpace (). add () code is expected to add that rigid body to the dynamics world.
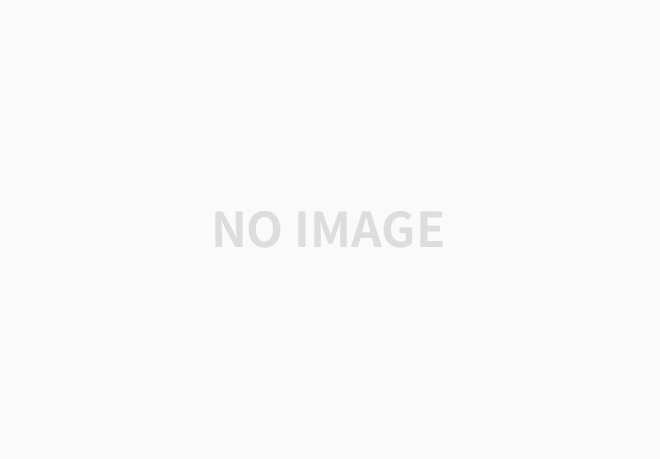
'Etc' 카테고리의 다른 글
개인 정보 처리 방침 (0) | 2020.06.13 |
---|---|
Jmonkey into Intellij (for android development) (0) | 2019.12.04 |
adMob - reward, libgdx (0) | 2019.11.30 |
adMob - interstitial, libgdx (0) | 2019.11.30 |
adMob - banner, libgdx (0) | 2019.11.23 |
댓글